What is the Web Storage API?
Whenever you're creating applications, sometimes you'll want to save certain chunks of data. Before HTML5,
data was usually stored in cookies. Nowadays, the Web Storage API exists, which is faster and more secure. The
Web Storage API is a set of mechanisms that allows you to store and retrieve key/value pairs. It contains two
mechanisms: sessionStorage
and localStorage
.
So when should you use which?
To put it simply, sessionStorage
data only remains for that specific session;
once you close out of the browser, the data is gone. localStorage
works differently —
the data persists even after the browser is closed. The only way to remove it is by clearing the browser cache.
Ideally, the Web Storage API should only be used for storing publicly available information. Anything private or sensitive should NEVER be stored on the client-side.
Storing and retrieving data
The syntax for using sessionStorage
and localStorage
is exactly the same, so for the sake of simplicity I'll be using localStorage
in
these examples. There's a few methods that both of these mechanisms come with:
setItem()
: Sets a key/value pair.getItem()
: Retrieves a key/value pair.removeItem()
: Removes a key/value pair.clear()
: Clears the entire storage.
To store an item in localStorage
, give it a key and a value:
localStorage.setItem('favoriteColor', 'green');
...this stores an item favoriteColor
with the value of green
.
We can see the item added to the Web Storage API by opening the developer console on Chrome > Application
> Storage > Local Storage...
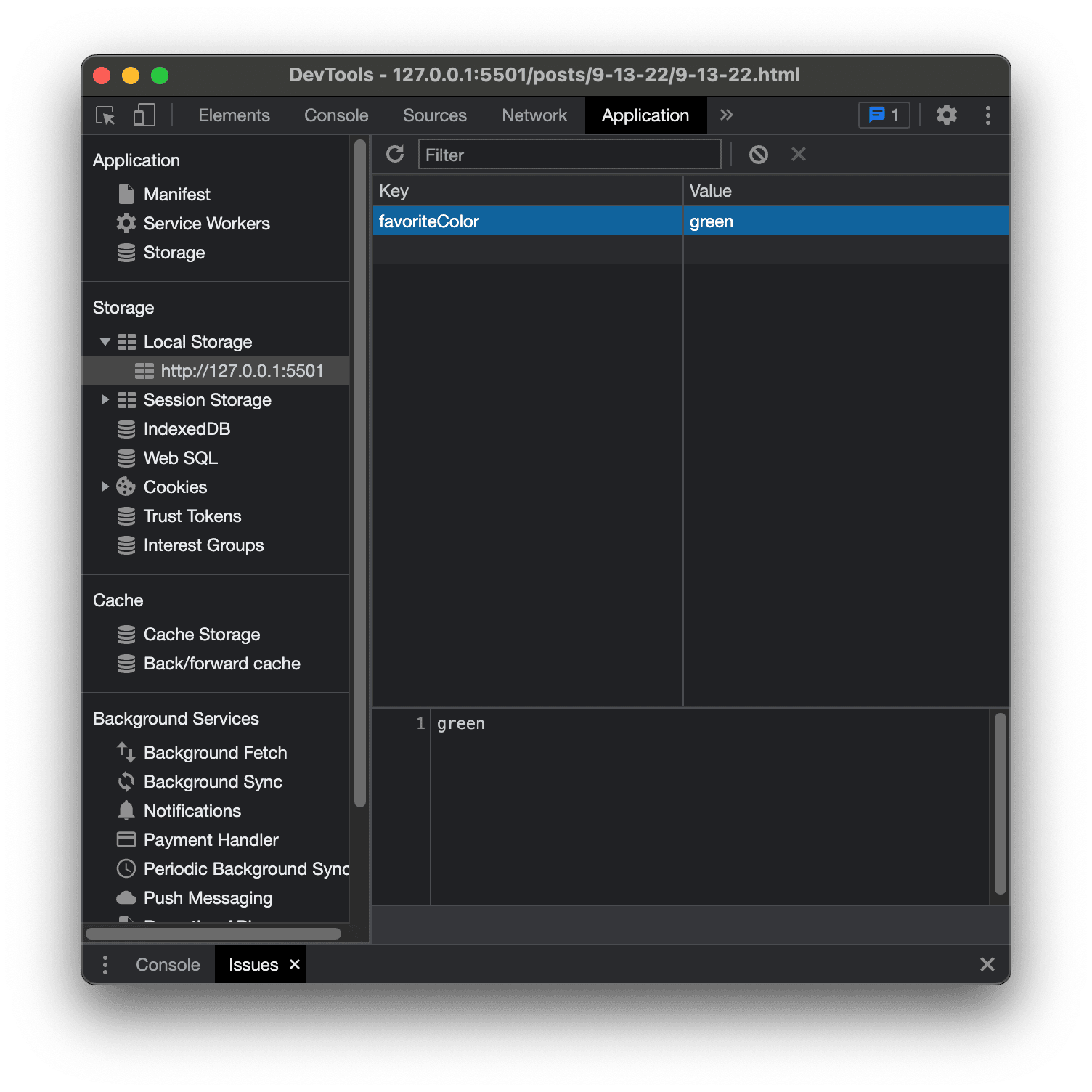
...the item can be retrieved by using the getItem()
method and storing it inside
a variable...
const cat = localStorage.getItem('favoriteColor');
console.log(cat);
// green
...removing favoriteColor
from localStorage
is
easy too...
localStorage.removeItem('favoriteColor');
...now if you check the browser's web storage again, you'll see that it's empty. Finally, using
localStorage.clear()
will remove every item from storage.
Storing objects
By default, both sessionStorage
and localStorage
only allow string values, but this doesn't mean that storing objects is impossible. If you're familiar with JSON,
then you should know JavaScript comes with two methods, JSON.parse()
and
JSON.stringify()
.
JSON.parse()
is used for turning JSON data to JavaScript objects, while
JSON.stringify()
is used for turning JavaScript objects to strings. Let's say
you have an array full of grocery items that you want to store:
const items = ['apples', 'bread', 'milk', 'cheese'];
...use JSON.stringify()
with setItem()
to store
this array as a string...
localStorage.setItem('groceries', JSON.stringify(items));
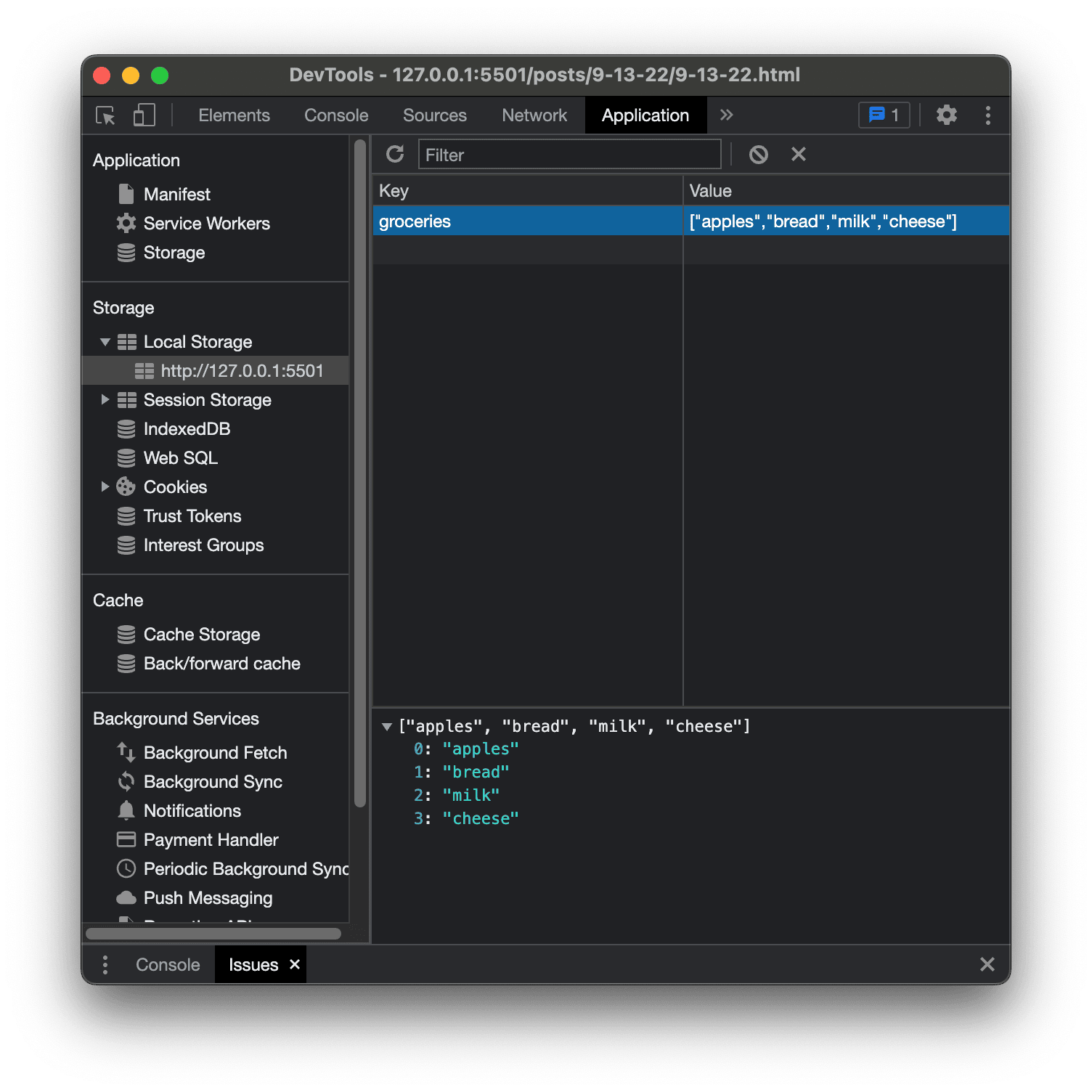
...the array is saved in storage as a string, but what if we wanted to add a few more items to the array? Well, since we can't directly mutate the stringified array, it must be converted back to an object...
const groceriesArray = JSON.parse(localStorage.getItem('groceries'));
...now just add the new items by destructuring all the elements into a new array, and convert it back to a string...
const groceriesArray = JSON.parse(localStorage.getItem('groceries'));
const newGroceries = ['corn', 'pasta'];
const updatedArray = [...groceriesArray, ...newGroceries];
localStorage.setItem('groceries', JSON.stringify(updatedArray));
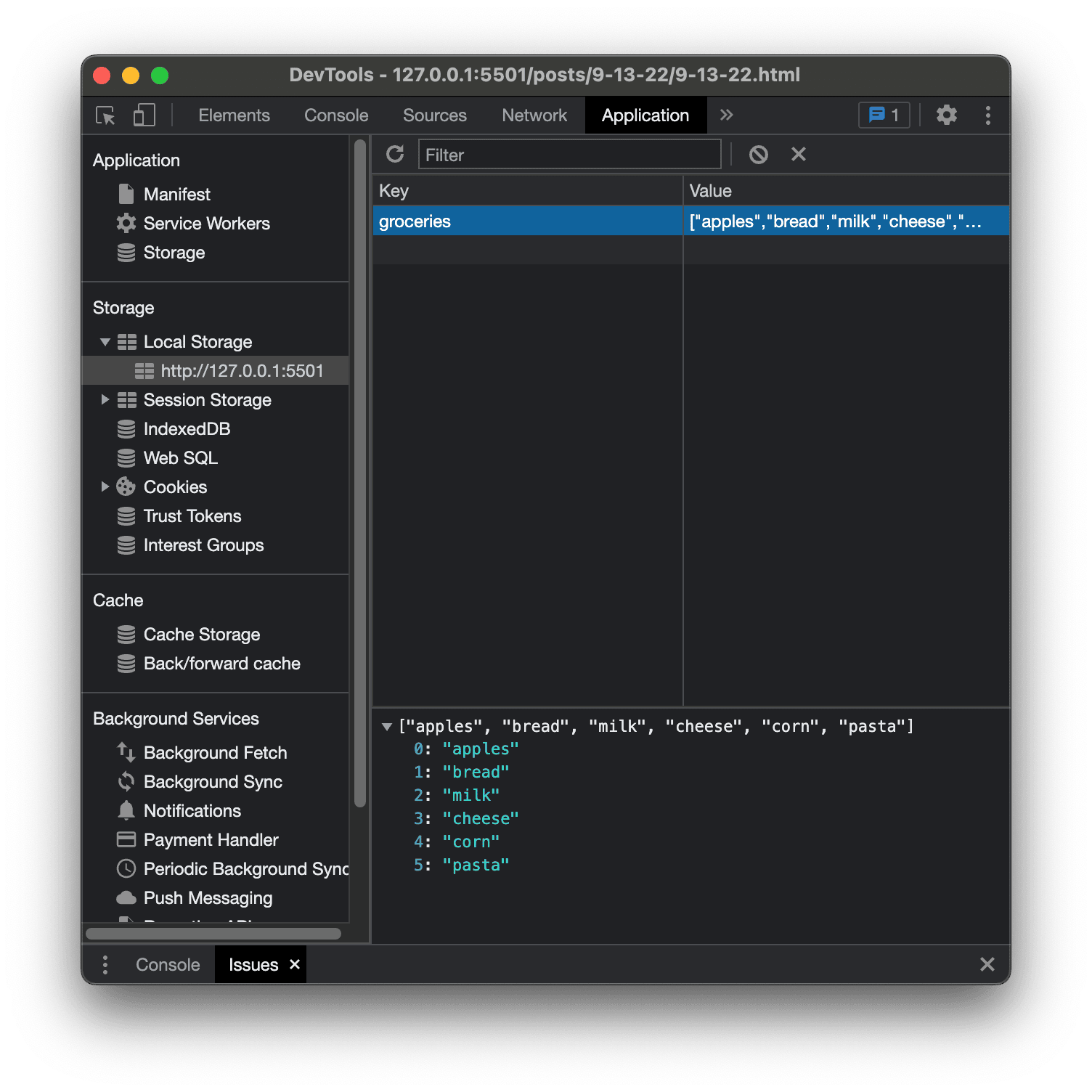
...and that's all there is to it! Remember, you should only use the Web Storage API for storing public information. NEVER store private or sensitive data on the client-side, as anyone has access to it.